Understanding JavaScript Closures: Unlocking the Secrets of Scope
- Suraj Kumar
- Mar 12
- 3 min read
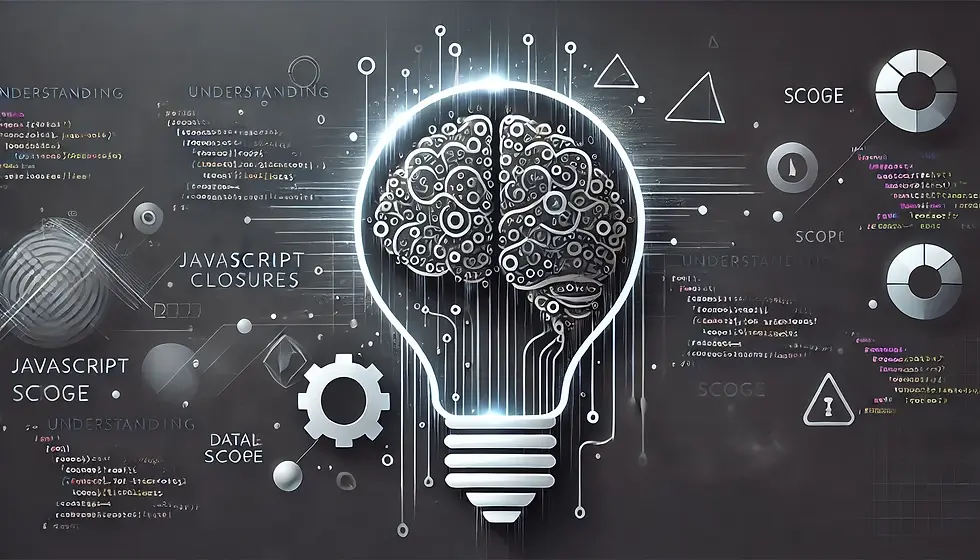
Introduction to Closures
In the world of JavaScript, closures are like hidden treasures—powerful yet often misunderstood. If you’ve ever scratched your head wondering why certain variables seem to "remember" their values even after a function has finished executing, you’ve encountered a closure. Let’s demystify this concept in simple terms and explore why mastering closures is crucial for any JavaScript developer.
What Are JavaScript Closures?
A closure occurs when a function "remembers" the variables from its outer scope even after that outer function has finished running. Essentially, closures give functions a memory, allowing them to retain access to variables defined outside their scope.
Simple Example:
function outerFunction() {
let count = 0; // Outer scope variable
return function innerFunction() {
count++;
console.log(count);
};
}
const counter = outerFunction();
counter(); // 1
counter(); // 2
counter(); // 3
Why does this work?
innerFunction still has access to the count variable, even though outerFunction has already finished executing. This is the essence of a closure!
Understanding Scope in JavaScript
Before diving deeper, let’s clarify scope—the foundation for closures.
Global Scope: Variables declared outside any function are in the global scope and can be accessed anywhere.
Local (Function) Scope: Variables declared inside a function are only accessible within that function.
Lexical (Static) Scope: JavaScript uses lexical scoping, meaning inner functions can access outer functions’ variables, but not vice versa.
Example:
let globalVar = "I am global";
function outer() {
let outerVar = "I am outer";
function inner() {
console.log(globalVar); // Accessible
console.log(outerVar); // Accessible
}
inner();
}
outer();
How Closures Work
Closures happen when:
An inner function is defined inside an outer function.
The inner function retains access to variables from its parent’s scope.
The outer function returns the inner function.
Think of closures as backpacks that inner functions carry, filled with variables from their outer scopes.
Real-World Use Cases of Closures
1. Data Privacy (Encapsulation):
Closures help simulate private variables—common in object-oriented programming but absent in JavaScript.
function createCounter() {
let count = 0; // Private variable
return {
increment: function() {
count++;
console.log(count);
},
getCount: function() {
return count;
}
};
}
const counter = createCounter();
counter.increment(); // 1
counter.increment(); // 2
console.log(counter.getCount()); // 2
2. Event Handlers and Callbacks:
Closures are fundamental in asynchronous JavaScript—used in event handlers, setTimeout, and Promises.
function addClickHandler() {
let count = 0;
document.getElementById('button').addEventListener('click', function() {
count++;
console.log(`Button clicked ${count} times`);
});
}
addClickHandler();
3. Function Factories:
Closures allow you to generate functions dynamically.
function multiplier(factor) {
return function(number) {
return number * factor;
};
}
const double = multiplier(2);
console.log(double(5)); // 10
const triple = multiplier(3);
console.log(triple(5)); // 15
Common Pitfalls and Debugging Closures
Pitfall: Using var inside loops
for (var i = 1; i <= 3; i++) {
setTimeout(function() {
console.log(i); // 4, 4, 4
}, 1000);
}
Why does this happen?
var has function scope—so all timeout callbacks reference the same i.
Fix: Use let (which has block scope):
for (let i = 1; i <= 3; i++) {
setTimeout(function() {
console.log(i); // 1, 2, 3
}, 1000);
}
Hands-On Example: Building a Counter with Closures
function createCounter() {
let count = 0;
return function() {
count++;
console.log(`Counter: ${count}`);
};
}
const myCounter = createCounter();
myCounter(); // Counter: 1
myCounter(); // Counter: 2
Explanation:
count is a private variable, only accessible via the returned inner function.
Each time myCounter is called, count retains its value.
Conclusion
Mastering JavaScript closures is like unlocking a new superpower in your coding journey. They enhance data privacy, enable dynamic function creation, and fuel async programming. By understanding scope and lexical environments, you’re better prepared to tackle advanced concepts in JavaScript.
Next Steps:
Experiment with closures in real-world scenarios.
Explore how closures interact with Promises and async functions.
Keep building and debugging!
Would you like us to add visual diagrams, or perhaps an interactive CodePen demo? Let me know in comments.
Comentarios