Resolving the 'CORS Policy Error' in Web Development: A Beginner's Guide
- Suraj Kumar
- Mar 2
- 2 min read
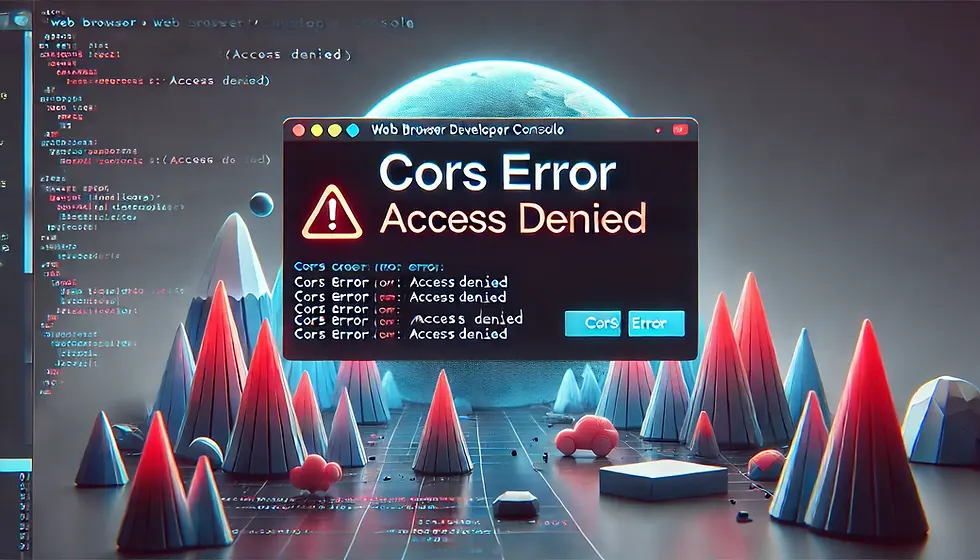
Introduction
If you've ever worked on a web development project involving APIs, you've likely run into the dreaded CORS policy error. Seeing something like this in your browser console can be frustrating:
Access to fetch at 'https://example.com/api' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
For new developers, this can be confusing — but don’t worry! In this guide, we’ll break down what CORS is, why the error happens, and how to fix it step by step.
What is CORS?
CORS stands for Cross-Origin Resource Sharing. It’s a security feature implemented by web browsers to prevent malicious websites from accessing data on other domains without permission.
Example:
If your website (running on http://localhost:3000) tries to fetch data from an API hosted at https://example.com, the browser blocks the request unless the server explicitly allows it.
Think of it like a security guard stopping a stranger (another domain) from entering a building (your site’s data) without proper authorization.
Why Does the CORS Error Happen?
The CORS error occurs when the server you're trying to fetch data from doesn’t include the correct headers to permit cross-origin requests. Specifically, the server needs to send the Access-Control-Allow-Origin header in its response.
Allowed:
Access-Control-Allow-Origin: *
or
Access-Control-Allow-Origin: http://localhost:3000
Blocked: If the server omits this header, the browser blocks the request, triggering the CORS error.
How to Fix the CORS Error
Here’s a step-by-step approach to resolve the issue:
1. If You Control the API (Backend Solution)
Add the correct CORS headers to your server’s response.
Node.js (Express) Example:
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors());
app.get('/api', (req, res) => {
res.json({ message: 'CORS enabled!' });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
You can customize the origins like this:
app.use(cors({ origin: 'http://localhost:3000' }));
2. If You Don’t Control the API (Frontend Solution)
Use a proxy server to make the request appear as if it's coming from the same origin.
React (package.json setup):
"proxy": "https://example.com"
Then fetch the API like this:
fetch('/api')
.then(response => response.json())
.then(data => console.log(data));
3. For Quick Testing (Browser Extension)
There are browser extensions like Allow CORS: Access-Control-Allow-Origin for testing purposes, but they should never be used in production as they bypass security measures.
4. Using a Cloud Function or Serverless Proxy
If the API doesn’t support CORS and you can't control it, set up a simple cloud function (on AWS Lambda, Netlify, or Vercel) to relay requests.
Best Practices
Never disable CORS permanently — it's a crucial security feature.
Whitelist specific domains instead of using Access-Control-Allow-Origin: * in production.
Test thoroughly after any CORS configuration changes.
Conclusion
The CORS policy error might seem like a tricky roadblock at first, but once you understand the basics, fixing it becomes straightforward. As a developer, mastering CORS will make working with APIs much smoother.
At Modelodge, we simplify complex development concepts like this — helping new developers level up their skills. Stay tuned for more practical guides and solutions in our Dev section!
Got a CORS issue you can’t crack? Drop a comment below or reach out to us — we’re here to help!
Comments